Java Tic Tac Toe Program

Website Development Services JavaTpoint (SSS IT Pvt Ltd) provides website development services in affordable cost. We develop websites on WordPress, Core PHP, CakePHP, CodeIgnitor, Open Cart, Servlet-JSP, Struts 2 and Spring technologies. Send us your requirement at hr@javatpoint.com. We will show you a free demo first, then we will proceed further. Personal Blog $150 Only School Website $200 Only Company Website $300 Only Showroom Website $300 Only News Portal $400 Only E-Commerce Website $500 Only Multivendor E-Commerce (PHP) $3000 Only Multivendor E-Commerce (Java) $18000 Approx.
Click on the Image to run the Applet Demo (tested on FireFox/Windows only) Let's Start with a 2-Player Console Non-OO Tic-Tac-Toe.
This Instructable will guide you, step by step, through making Tic Tac Toe in Java! This is not intended to be an overview of the Java language, but more of a guided example. The first step will go over some basic concepts to make the rest of the guide go down smoother. All other aspects will be briefly explained as they’re introduced. You need to be competent with a computer and your chosen operating system. You do not need any prior exposure to Java or any other programming language.
However, this guide may go over a bit too much, too fast, and not deep enough for some people. If you find yourself getting confused often, take the time to read over a Java overview. For this Instructable, the main thing you need to know about Java is that it is an object-oriented programming language. An object-oriented programming language consists of objects that communicate with each other. Objects contain associated data fields and typically have methods that perform a variety of tasks. These tasks vary from manipulating the object’s data to communicating with other objects. To break this down, a Java program is typically comprised of objects.
These objects are defined by classes, and within these classes are instance variables and methods. Classes These are what you will be coding.
Think of classes as the blueprints for the object. They describe what the object contains and what it can do. Instance Variables These are the attributes of the object. For example, a Student object would have instance variables like name, GPA, and classification. Methods These are the actions that an object can make. To use the Student example, these could be things like getting the GPA or taking a class.
Don’t worry if you don’t quite grasp these concepts yet. Building the program will help make these more concrete. First, you need to download and install the Java Development Kit (JDK), which will provide you the backend tools for developing. This can be found. Once you load that page up, download and install the JDK. For help, follow these: Note: The number in parentheses signifies its location on the image above.
Click on the Download button (1) underneath JDK. Accept the license agreement. Click on the link (4) that that corresponds with your operating system (3). Once the file is down downloading, install it. Eclipse is the program you will be programming in. Now, you don’t install Eclipse, so follow the instructions.
Look for Eclipse IDE for Java Developers. If you have Windows, download from the correct link on the right. If you do not have Windows, click on Details. (3) Then choose the correct download link on the right.
Once you get to the download page, click on the green down arrow and download Eclipse. This may take a while, as Eclipse is rather big. After it’s done, find where you saved. Extract the zip file to wherever you want.
I put it in my Programs folder. Shortly after you start up Eclipse, it'll ask you for a workspace location. Put this wherever you want. After you choose, the Eclipse workbench will pop up. If it doesn't, click on the arrow on the right side. Take a few minutes to play around and familiarize yourself with the interface. The Files For this project, I wrote most of the code for you.
To get it set up in your Eclipse, follow this: 1. Download the. Go to File - Import.
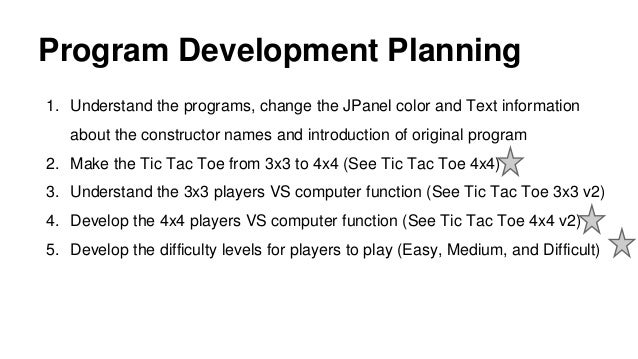

Drill down the General folder (1) and select Existing Projects into Workspace. (2) Click Next. Click Select archive file. Click Browse. (3) and navigate to where you saved the zip file. Click Finish.
Packages (1) Packages provide an organizational tool for Java projects by grouping similar classes together. A common naming convention is to use your domain backwards.
As you can see, this project uses the Instructable domain. This helps to eliminate any issues when integrating Java programs. You may not run into this, but it is a good habit to get into. The two packages in this project are game and main. The game package contains the more backend information for running the game. The main package includes the class that interacts with the user.
Classes (2) There are three classes in this project-Board, Player, an Runner. When deciding which classes need to be made for a project, think about the elements of what you're making. In this case, Tic Tac Toe only had two notable elements-a player to play the game and a board to play the game on.
Tic Tac Toe Java Program Source Code
More complex programs will require more classes. Board This represents the Tic Tac Toe board. This class handles keeping track the of the state of the board and if the board is a winning board.
Player This represents a player in Tic Tac Toe. It's a fairly simple class. It stores the player's name and it's playing symbol It also handles marking the board. Runner This class is what runs the program. We'll go into an overview in the next step.
Let's look over the Runner class and try to get a feel for how it's structured. Class Declaration Look toward the top where it says public class Runner. This is the class declaration. The public part is an access modifier. An access modifier says who has access to this class. Access modifiers can be: - Public - Protected - Private You will almost always make a class public. Protected and private are reserved for special types of classes that we will not get into.
Just know that public classes can be used by anything. The second part of the declaration-class-says we're making a class. The third part-Runner-is the name. The curly brace starts a code block. All code for this class must be within the two curly braces. Note: Curly braces are used for all code blocks, not just classes. Main Method The main method, as noted in the picture, is what runs when you run the program.
Don't worry about the whole public static void mess. But, do take note as to what's happening inside the main method. The first line creates a Board object called board. The rest of the lines are method calls.
Method calls run the method with the given name. Typical main methods contain more code than this, but I organized it this way for a clearer understanding of the process the program goes through.
Method Headers Method headers are the first line of methods. They describe what the method intends to do.
Let's look at private static String playGame(Board board) private is an access modifier, just like the class one. In this case, private means that only the Runner class can call this method. Ignore static.
This is more advanced topic. String describes what the method will give back once it finishes running. In this case, it’s a String, which means it’ll give back a word or a string of words. In this case, it's returning null, which represents nothing.
PlayGame is simply the name of the method. (Board board) is called a parameter. This is outside information that the method needs to run. In this case, it needs a Board object. You will be doing most of the work in the playGame method. First, we need to set up some necessary objects.
Every game needs players. In the case of Tic Tac Toe, we need two players. Delete the TODO tag and paste in the following: Player playerX = new Player('X', board); Player playerO = new Player('O', board); These lines declare and set two player objects. One has a symbol of X and the other a symbol of O. They also give the player objects the board that they will play on. Next we need to set up a way of taking input from the user.
To do this, paste the following after the players: Scanner in = new Scanner(System.in); This sets up a Scanner object called in. System.in simply means we’re taking input from the console.
If you see warnings, don't worry. They're supposed to be there. We need to get the user's input as to where they want to make a move. This is where we use the Scanner object we made earlier.
However, first we need to make a variable to store the input in. Paste this outside of the loop: int play; Next, paste this after the if statement: System.out.print(currPlayer.getName + ', make a move (1-9): '); play = in.nextInt; There's a lot going on here. First, we print out a prompt for the user to input something. System.out.println prints whatever is inside its parentheses to the console. This prints the current player’s name followed by “make a move (1 – 9)”.
Let’s stop a second at currPlayer.getName. This is still a method call, just like the ones in the main method. However, in this case we’re calling it on an object. There is a method inside of the Player object called getName, and we’re using that to retrieve the player’s name.
After that, we have the act of taking in the input. To take in integer input, we use the nextInt method of the Scanner object. Then we store this input in our play variable. Now that we have the play the player wants to make, we need to actually make it. Remington model 580 serial numbers. The way I wrote the code, after a play is made on the board, it determines if that play was a winning one.
So, we need to make a variable to store if a win has occurred. Outside the loop, paste this: boolean hasWon = false; This is set to false, as there is not a win until it happens.
Next, we need to call the right method to make the play. Paste this after the input: hasWon = currPlayer.makePlay(Player.cellsplay - 1); This takes whatever the makePlay method of Player returns and stores it in hasWon. It will return true if the play won the game, false otherwise. Player.cellsplay - 1 figures out which cell the player wants to play in.
Don't worry about the technicalities of it.